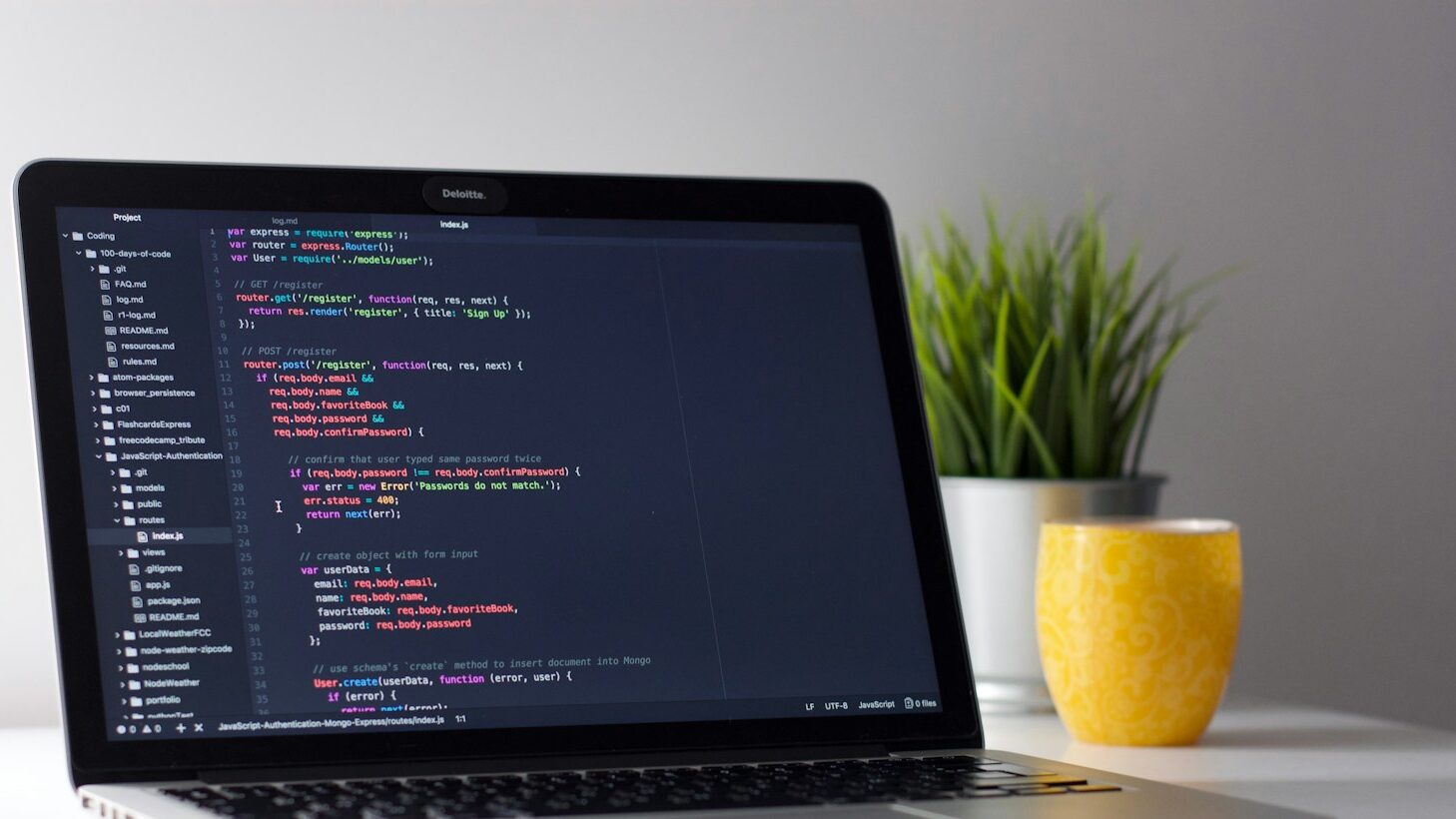
Asynchronous programming allows your app to complete time-consuming tasks, such as retrieving an image from the web, or writing some data to a web server, while running other tasks in parallel and responding to the user input. This improves the user experience and the overall quality of your software.
What Is Asynchronous Programming
Let’s use a simple story to explain asynchronous programming in Flutter. Imagine you’re running a small café and you have a few tasks to manage: making coffee, baking cookies, and cleaning tables. You want to get everything done efficiently without keeping your customers waiting.
If you try to do everything yourself, one after the other, it might look like this:
- Make Coffee: You start making a cup of coffee. This takes 5 minutes.
- Bake Cookies: After finishing the coffee, you start baking cookies. This takes 15 minutes.
- Clean Tables: Once the cookies are done, you start cleaning the tables. This takes 10 minutes.
So, the total time taken is 5 + 15 + 10 = 30 minutes.
During this time, your customers have to wait a long time for their orders, and your café isn’t running smoothly.
Now, imagine you have three helpers. You can assign each task to a helper, and they can work at the same time:
- Helper 1 makes coffee.
- Helper 2 bakes cookies.
- Helper 3 cleans the tables.
This way, all tasks happen simultaneously. The longest task (baking cookies) takes 15 minutes. So, instead of 30 minutes, everything is done in 15 minutes.
Asynchronous Programming in Flutter
In Flutter, when you have tasks like fetching data from the internet, you don’t want your app to freeze and make users wait. Instead, you can handle these tasks in the background while the app continues to work smoothly.
Key Concepts
- Future (Helper Promise): A Future in Flutter is like a promise from a helper. It means, “I’ll do this task and let you know when it’s done.”
- async and await (Asking Helpers): When you use async and await, it’s like telling a helper to do a task (async) and then waiting for them to finish (await) before moving on to the next step.
- Stream (Ongoing Tasks): A Stream is like a task that produces multiple results over time, like a customer who keeps ordering more coffee every few minutes.
Imagine your Flutter app needs to get the latest news from the internet. Instead of making the user wait (like you doing all tasks alone), it asks a helper (Future) to get the news.
Future<String> fetchNews() {
// Simulate fetching news, which takes 5 seconds
return Future.delayed(Duration(seconds: 5), () => 'Here is the latest news!');
}
void main() async {
print('Fetching news...');
var news = await fetchNews(); // Wait for the helper to finish
print(news); // Show the news to the user
}
In this example:
Future<String> fetchNews()
is like assigning the news fetching task to a helper.await fetchNews(
) is like waiting for the helper to finish fetching the news.- While waiting, the app can continue doing other things, just like you would continue managing the café.
Widgets for Helpers in Flutter
Flutter provides special widgets to work with these helpers:
- FutureBuilder: This widget helps you work with a Future. It’s like having a display that updates when the helper finishes the task.
- StreamBuilder: This widget helps you work with a Stream. It updates every time a new piece of information arrives, like a helper bringing fresh orders to the counter.
Conclusion
Asynchronous programming in Flutter is like managing a café with helpers. You assign tasks to helpers (use Futures and Streams), and while they are working, you continue with other tasks. This makes your app (or café) run smoothly and efficiently, keeping everyone happy and satisfied.
Leave a Reply